Solving the Java Deserialization Threat: From Bytes to Breaches
Explore deserialization risks, common attack vectors, and how GRSee Consulting’s tailored strategies help protect applications from evolving threats.
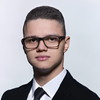
Updated February 25, 2025.
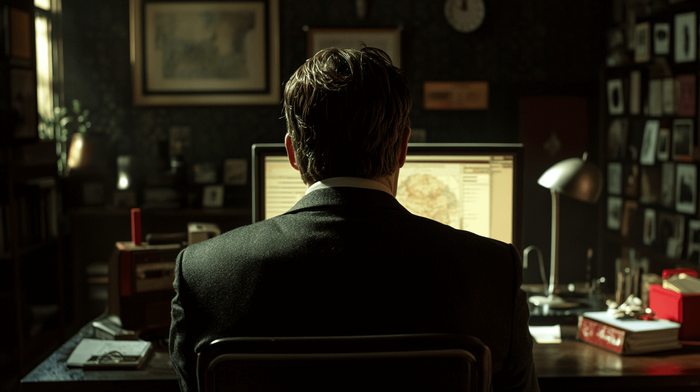
Java deserialization is a fundamental process in applications, enabling serialized data to be transformed back into usable objects. While essential for data storage and communication, it has also become a major security concern. Vulnerabilities in deserialization have led to attacks such as remote code execution (RCE) and unauthorized access, emphasizing the need for secure coding practices as highlighted in the OWASP Top 10 Proactive Controls 2024.
The risks of insecure deserialization continue to grow, exposing sensitive data and critical operations to threats. Attackers exploit poorly configured deserialization processes to manipulate application behavior or deploy malicious payloads. In this article, we'll delve into Java deserialization, its risks, and strategies to safeguard enterprise applications from these vulnerabilities.
» Ready to enhance your security? Let our experts help you
What Is Deserialization in Java?
Serialization converts an object into a byte stream for easy storage or transmission, preserving object states and enabling data exchange between systems. Serialized objects can be sent over networks, stored or uploaded in files, or saved in databases, ensuring they can be recreated when needed.
Deserialization works inversely, reconstructing objects from a byte stream and restoring them to a usable form. It is critical for maintaining consistency in distributed systems and supports functionalities like real-time updates. However, insecure deserialization in Java can expose applications to security risks, such as payload injections or object manipulation.
Serialization and deserialization enable seamless data exchange and object restoration, but insecure processes can pose significant security risks to applications.
» Understand the importance of risk assessment
Example: How Deserialization Works in Java
For an object to be deserialized, it must implement the java.io.Serializable interface. This ensures that the object’s class adheres to specific methods required for the serialization and deserialization processes. Primarily, the class relies on two methods:
Method 1
writeObject(java.io.ObjectOutputStream)
This handles the serialization process, converting the object into a byte stream for storage or transmission.
Method 2
readObject(java.io.ObjectInputStream)
This manages the deserialization process, reconstructing the object from the byte stream.
Simple Code Example
import java.io.*;
class Example implements Serializable {
String name = "John";
}
public class Main {
public static void main(String[] args) throws Exception {
try (ObjectOutputStream out =
new ObjectOutputStream(new FileOutputStream("data.ser"))) {
out.writeObject(new Example());
}
try (ObjectInputStream in =
new ObjectInputStream(new FileInputStream("data.ser"))) {
System.out.println(((Example) in.readObject()).name);
}
}
}
Output:
John
Role of Deserialization in Enterprise Applications
Deserialization is essential in enterprise applications, enabling serialized data streams to be converted back into objects in memory. Developers rely on this functionality when it meets application needs and constraints, ensuring data can be efficiently stored, transmitted, and reconstituted for seamless functionality.
- Persistent storage: Enables applications to reconstruct data stored in serialized formats, allowing objects to be accessed and manipulated as they were originally saved. This is essential for long-term data management and archival processes.
- Inter-application communication: Facilitates seamless data exchange between different systems or services by sharing objects, ensuring interoperability across platforms in distributed architectures.
- Restoring application states: Allows applications to recover objects after crashes or restarts, ensuring continuity and reliability. This enables systems to resume operations without losing critical data or functionality.
Why Is Java Deserialization Vulnerable?
Java deserialization, while integral to many enterprise applications, presents significant security challenges. Its primary vulnerability lies in how deserialization processes inherently trust incoming data.
If this data is manipulated or maliciously crafted, it can lead to severe exploits, compromising application integrity and security.
Key Risks
- Remote code execution (RCE): Attackers can craft serialized data to execute arbitrary code on the target system during deserialization. This exploit is particularly dangerous because it allows full control over the affected application or system. RCE vulnerabilities have been at the center of numerous high-profile security breaches.
- Injection attacks: Deserialization flaws often enable attackers to inject malicious payloads, manipulate application behavior, or steal sensitive data. These payloads can be designed to bypass security controls, disrupt operations, or extract sensitive information from the system.
- Gadget chains: Attackers exploit existing classes in an application to create "gadget chains" that execute malicious operations during deserialization. These chains often leverage legitimate functionality in unintended ways, enabling attackers to bypass security measures and carry out complex attacks.
» Make sure you know how to fortify your business against password spraying attacks
Notable CVEs Highlighting Deserialization Vulnerabilities
- CVE-2013-2165: In Red Hat JBoss RichFaces versions 3.x through 5.x, the ResourceBuilderImpl.java component does not restrict the classes for which deserialization methods can be called. This lack of restriction allows remote attackers to execute arbitrary code via crafted serialized data.
- CVE-2016-6497: The Groovy LDAP API in Apache, specifically the LDAP.java file, permits LDAP entry poisoning attacks. Attackers can exploit this by setting the returnObjFlag to true for all search methods, leading to potential security breaches.
- CVE-2020-9496: Apache OFBiz version 17.12.03 is vulnerable due to unsafe deserialization and Cross-Site Scripting (XSS) issues in its XML-RPC requests. These vulnerabilities can be exploited by remote attackers to execute arbitrary code or conduct XSS attacks.
Recognizing and Detecting Vulnerabilities
Detecting deserialization vulnerabilities in Java applications involves understanding the system's architecture and using advanced tools. These issues often occur when serialized objects from untrusted sources are deserialized without proper validation, especially in frameworks relying heavily on client-server object serialization.
Common Indicators of Vulnerability
- The application accepts serialized objects from untrusted sources, such as user input or external systems
- The use of frameworks or libraries that automatically deserialize objects without explicit developer oversight
- The presence of classes implementing readObject() without proper input validation or checks on the source of the input stream
Tools and Techniques for Detection
- Static analysis tools: These tools perform vulnerability scans on codebases to identify classes using readObject() and track the origin of input streams to locate potential vulnerabilities.
- Threat modeling: Helps identify potential attack vectors by analyzing the application's data flow and identifying areas where untrusted data interacts with deserialization processes.
- Dynamic testing: Simulates deserialization attacks to evaluate whether the application is susceptible to exploits.
- Penetration testing: Mimics real-world attacks to assess the effectiveness of current deserialization safeguards.
» Secure your systems effectively—Learn about the different types of penetration testing
Best Practices for Secure Deserialization
Ensuring secure deserialization in Java applications involves adopting robust practices that minimize risk exposure. Since the Java Serializable interface can introduce vulnerabilities when handling untrusted input, alternative methods and safeguards should be prioritized.
- Avoid java.io.Serializable for untrusted input: This interface is prone to exploitation and should not be used to handle data from untrusted sources.
- Deserialization filters (Java 9+): Implement whitelists to define which classes are allowed during deserialization. These filters inspect incoming objects before they are deserialized, effectively preventing unauthorized objects from being processed.
- Custom serialization methods: Carefully implement serialization methods to restrict the types of objects allowed for deserialization in Java.
Best Practice | Implementation | Outcome |
---|---|---|
Avoid Serializable | Use alternative serialization methods | Reduces risk of arbitrary deserialization |
Apply deserialization filters | Whitelist allowed classes during deserialization | Blocks malicious objects during runtime |
Custom serialization methods | Restrict the types of objects allowed for deserialization | Limits deserialization to safe objects |
Whitelisting and Blacklisting Strategies
Whitelisting ensures that only explicitly permitted objects are deserialized in Java, reducing attack surfaces. However, the approach has limitations:
- Exploitable gadget chains might still exist if allowed objects are improperly defined
- Maintaining accurate and updated whitelists can be challenging in large, evolving systems
Blacklisting, while less effective, might leave room for attackers to exploit objects not explicitly blocked. A combination of strategies can provide a more secure implementation.
» Boost your organization's security with the CIA triad
Advanced Strategies for Mitigation
Sandboxing and Containerization
These techniques create isolated environments that contain exploits, preventing them from spreading to other parts of the application. By restricting access to external resources and adding complexity to post-exploitation steps, sandboxing and containerization minimize the impact of vulnerabilities.
Regular Security Audits and Penetration Testing
Comprehensive audits and penetration tests uncover deserialization vulnerabilities and assess their potential impact. They provide actionable insights and ensure that security measures remain effective against evolving threats.
» Want to implement penetration testing? Here are the pentest steps to follow
Keeping Libraries and Dependencies Updated
Regular updates to libraries and frameworks address known vulnerabilities, reducing the risk of exploitation. Monitoring security advisories for third-party components helps maintain a secure software environment.
How GRSee Supports Your Security Goals
At GRSee Consulting, we bring extensive expertise in identifying and mitigating deserialization vulnerabilities. Our consultants focus on tailored strategies to strengthen your application security, leveraging advanced techniques like threat modeling, penetration testing, and secure coding practices. Our cyberservices don't just address vulnerabilities; we equip your teams with actionable recommendations to guard against evolving threats proactively.
Our approach has delivered measurable success across different industries. Understanding the unique requirements of your organization helps us ensure good compliance with industry standards while enhancing your overall security posture.
» Get started with GRSee today: Contact us